This was a pain.
As I didn’t want to hang around too long, I downloaded the minimal ISO for CentOS. I attempted to create a VM, but this failed when VMware tools were installing:
Installing VMware Tools, please wait...
mount: special device /dev/hda does not exist
mount: block device /dev/sr0 is write-protected, mounting read only
/etc/rc5.d/S99local: line 25: eject: command not found
/etc/rc5.d/S99local: line 25: eject: command not found
I had to create a new VM without an OS, then mount the ISO as the CD drive, reboot and install that way.
Then I had to somehow configure the network. I couldn’t install VMware tools because perl wasn’t installed, so I don’t know if that might have fixed it.
I ended up editing /etc/sysconfig/network-scripts/ifcfg-eth0, and I had to use VI because I couldn’t install nano… An issue I had was that I didn’t know what the gateway was – I had NAT networking setup in VMware workstation. There’s a handy tool to find out bundled with VMware Workstation. It also works with VMware player, but doesn’t come with it. I found it here:
C:\Program Files (x86)\VMware\VMware Workstation\vmnetcfg.exe
I selected the NAT network, then NAT settings, and this shows the gateway:
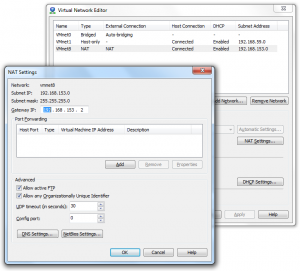
My /etc/sysconfig/network-scripts/ifcfg-eth0 ended as this:
DEVICE=eth0
HWADDR=00:0C:29:FC:8F:27
NM_CONTROLLED=no
ONBOOT="yes"
IPADDR=192.168.153.20
BOOTPROTO=none
NETMASK=255.255.255.0
GATEWAY=192.168.153.2
DNS1=8.8.8.8
DNS2=8.8.4.4
USERCTL=no
TYPE=Ethernet
The device, MAC and a few others were already set. The DNS entires I have used are Google’s DNS servers. The IP address I chose was a free one (all apart from .1 should be free, I think) on the network – select the DHCP settings for the network in the Virtual Network Editor to see the address range. I also updated the file /etc/sysconfig/network to this:
NETWORKING=yes
That file didn’t need the gateway, as I read elsewhere. I then restarted the network:
service network restart
I was then able to connect to the internet and install perl and then VMware tools. It all turned out to be a complete waste of time, but ah well.
Vmware Workstation->VM (to the right of File, Edit, View)->Install VMware Tools…
yum install perl
mkdir /mnt/cdrom
mount /dev/cdrom /mnt/cdrom
cd /tmp
tar -xzf /mnt/cdrom/VMwareTools-<version>.tar.gz
cd vmware-tools-distrib
./vmware-install.pl
[hit enter a lot to accept all the defaults]